I’ve just come back from a ten-day trip to Germany. The trip kicked off with Indie Web Camp Düsseldorf over the course of a weekend.
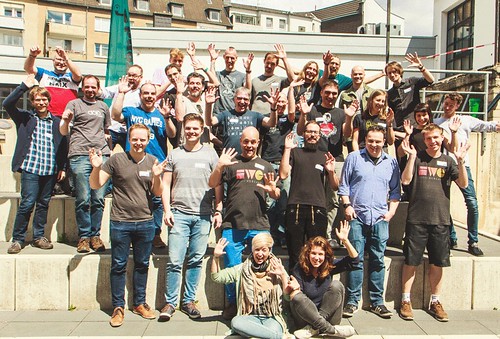
Once again the wonderful people at Sipgate hosted us in their beautiful building, and once again myself and Aaron helped facilitate the two days.
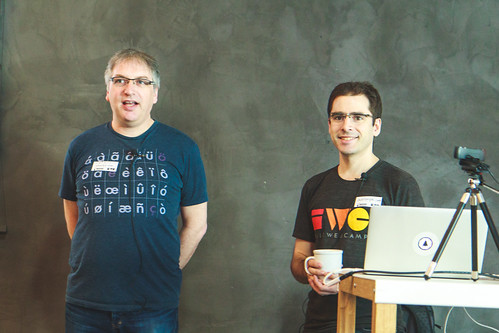
Saturday was the BarCamp-like discussion day. Plenty of interesting topics were covered. I led a session on service workers, and that’s also what I decided to work on for the second day—that’s when the talking is done and we get down to making.

I like what Ethan is doing on his offline page. He shows a list of pages that have been cached, but instead of just listing URLs, he shows a title and description for each page.
I’ve already got a separate cache for pages that gets added to as the user browses around my site. I needed to figure out a way to store the metadata for those pages so that I could then display it on the offline page. I came up with a workable solution, and interestingly, it involved no changes to the service worker script at all.
When you visit any blog post, I put metadata about the page into localStorage
(after first checking that there’s an active service worker):
if (navigator.serviceWorker && navigator.serviceWorker.controller) {
window.addEventListener('load', function() {
var data = {
"title": "A minority report on artificial intelligence",
"description": "Revisiting Spielberg’s films after a decade and a half.",
"published": "May 7th, 2017",
"timestamp": "1494171049"
};
localStorage.setItem(
window.location.href,
JSON.stringify(data)
);
});
}
In my case, I’m outputting the metadata from the server, but you could just as easily grab some from the DOM like this:
var data = {
"title": document.querySelector("title").innerText,
"description": document.querySelector("meta[name='description']").getAttribute("contents")
}
Meanwhile in my service worker, when you visit that same page, it gets added to a cache called “pages”. Both localStorage
and the cache
API are using URLs as keys. I take advantage of that on my offline page.
The nice thing about writing JavaScript on my offline page is that I know the page will only be seen by modern browsers that support service workers, so I can use all sorts of fancy from ES6, or whatever we’re calling it now.
I start by looping through the keys of the “pages” cache (that’s right—the cache
API isn’t just for service workers; you can access it from any script). Then I check to see if there is a corresponding localStorage
key with the same string (a URL). If there is, I pull the metadata out of local storage and add it to an array called browsingHistory
:
const browsingHistory = [];
caches.open('pages')
.then( cache => {
cache.keys()
.then(keys => {
keys.forEach( request => {
let data = JSON.parse(localStorage.getItem(request.url));
if (data) {
data['url'] = request.url;
browsingHistory.push(data);
}
});
Then I sort the list of pages in reverse chronological order:
browsingHistory.sort( (a,b) => {
return b.timestamp - a.timestamp;
});
Now I loop through each page in the browsing history list and construct a link to each URL, complete with title and description:
let markup = '';
browsingHistory.forEach( data => {
markup += `
<h2><a href="${ data.url }">${ data.title }</a></h2>
<p>${ data.description }</p>
<p class="meta">${ data.published }</p>
`;
});
Finally I dump the constructed markup into a waiting div
in the page with an ID of “history”:
let container = document.getElementById('history');
container.insertAdjacentHTML('beforeend', markup);
All those steps need to be wrapped inside the then
clause attached to caches.open("pages")
because the cache
API is asynchronous.
There you have it. Now if you’re browsing adactio.com and your network connection drops (or my server goes offline), you can choose from a list of pages you’ve previously visited.
The current situation isn’t ideal though. I’ve got a clean-up operation in my service worker to limit the number of items stored in my “pages” cache. The cache never gets bigger than 35 items. But there’s no corresponding clean-up of metadata stored in localStorage
. So there could be a lot more bits of metadata in local storage than there are pages in the cache. It’s not harmful, but it’s a bit wasteful.
I can’t do a clean-up of localStorage
from my service worker because service workers can’t access localStorage
. There’s a very good reason for that: the localStorage
API is synchronous, and everything that happens in a service worker needs to be asynchronous.
Service workers can access indexedDB
: it’s asynchronous. I could use indexedDB
instead of localStorage
, but I’m not a masochist. My best bet would be to use the localForage library, which wraps indexedDB
in the simple syntax of localStorage
.
Maybe I’ll do that at the next Homebrew Website Club here in Brighton.