-
-
Notifications
You must be signed in to change notification settings - Fork 2.6k
Home
Mariano Eduardo Rodriguez edited this page Nov 6, 2022
·
20 revisions
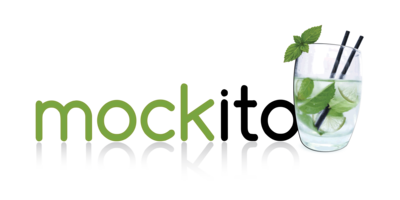
Mockito is a mocking framework for unit tests in Java. It has been designed to be intuitive to use when the test needs mocks.
- Simple usage : stub, use, verify
- Programatic creation of mocks via
mock()
orspy()
- Programmatic stubbing via
Mockito.when(mock.action()).thenReturn(true)
BDDMockito.given(mock.action()).willReturn(true)
- Customize mock answer or provide your own
- Programmatic verification via
Mockito.verify(mock).action()
BDDMockito.then(mock).should().action()
- Annotation sugar via
@Mock
,@Spy
,@Captor
or@InjectMocks
- JUnit first class support via the runner
MockitoJUnitRunner
and the now favored ruleMockitoJUnit.rule()
- Do not mock types you don't own
- Don't mock value objects
- Don't mock everything
- Show some love with your tests
// You can mock concrete classes and interfaces
TrainSeats seats = mock(TrainSeats.class);
// stubbing appears before the actual execution
when(seats.book(Seat.near(WINDOW).in(FIRST_CLASS))).thenReturn(BOOKED);
// the following prints "BOOKED"
System.out.println(seats.book(Seat.near(WINDOW).in(FIRST_CLASS)));
// the following prints "null" because
// .book(Seat.near(AISLE).in(FIRST_CLASS))) was not stubbed
System.out.println(seats.book(Seat.near(AISLE).in(FIRST_CLASS)));
// the following verification passes because
// .book(Seat.near(WINDOW).in(FIRST_CLASS)) has been invoked
verify(seats).book(Seat.near(WINDOW).in(FIRST_CLASS));
// the following verification fails because
// .book(Seat.in(SECOND_CLASS)) has not been invoked
verify(seats).book(Seat.in(SECOND_CLASS));
The BDD way :
// You can mock concrete classes and interfaces
TrainSeats seats = mock(TrainSeats.class);
// stubbing appears before the actual execution
given(seats.book(Seat.near(WINDOW).in(FIRST_CLASS))).willReturn(BOOKED);
// the following prints "BOOKED"
System.out.println(seats.book(Seat.near(WINDOW).in(FIRST_CLASS)));
// the following prints "null" because
// .book(Seat.near(AISLE).in(FIRST_CLASS))) was not stubbed
System.out.println(seats.book(Seat.near(AISLE).in(FIRST_CLASS)));
// the following verification passes because
// .book(Seat.near(WINDOW).in(FIRST_CLASS)) has been invoked
then(seats).should().book(Seat.near(WINDOW).in(FIRST_CLASS));
// the following verification fails because
// .book(Seat.in(SECOND_CLASS)) has not been invoked
then(seats).should().book(Seat.in(SECOND_CLASS));
- The very latest javadoc
- Release Notes
- If you have questions, please read our FAQ Hello, world!
- Read the features and motivation
- Want to contribute, read this
- Want to find online courses, visit this
- License
- Related Projects