The App GardenCreate an App API Documentation Feeds What is the App Garden? |
User Authentication
Many of Flickr’s API methods require the user to be signed in. In the past we were using our own authentication API, but now, users should only be authenticated using the OAuth specification which is the industry standard. By using the OAuth standard you will provide in your applications a secure way for people to sign-in into their Flickr accounts with all the different account types Flickr is supporting (Yahoo! ID, Google ID, Facebook). Flickr’s OAuth flows work for web-applications, desktop apps and mobile applications as well.
Using OAuth with Flickr
What is OAuth?
OAuth is an open, simple, and secure protocol that enables applications to authenticate users and interact with Flickr on their behalf. The end user's information is securely transferred without revealing the identity of the user. At Flickr, we use OAuth to verify that an application trying to interact with Flickr on your behalf is doing so only with the permissions you have granted it.
This document supplements the OAuth Core 1.0 Revision A specification and explains how the authorization process works with Flickr.
The simplest way to get started with OAuth is to use a library with your programming language. To find a library for your programming language, visit the OAuth Code page.
We also have a transition process in place for existing applications, using the old Auhentication API, to migrate to OAuth.
The OAuth flow has 3 steps:
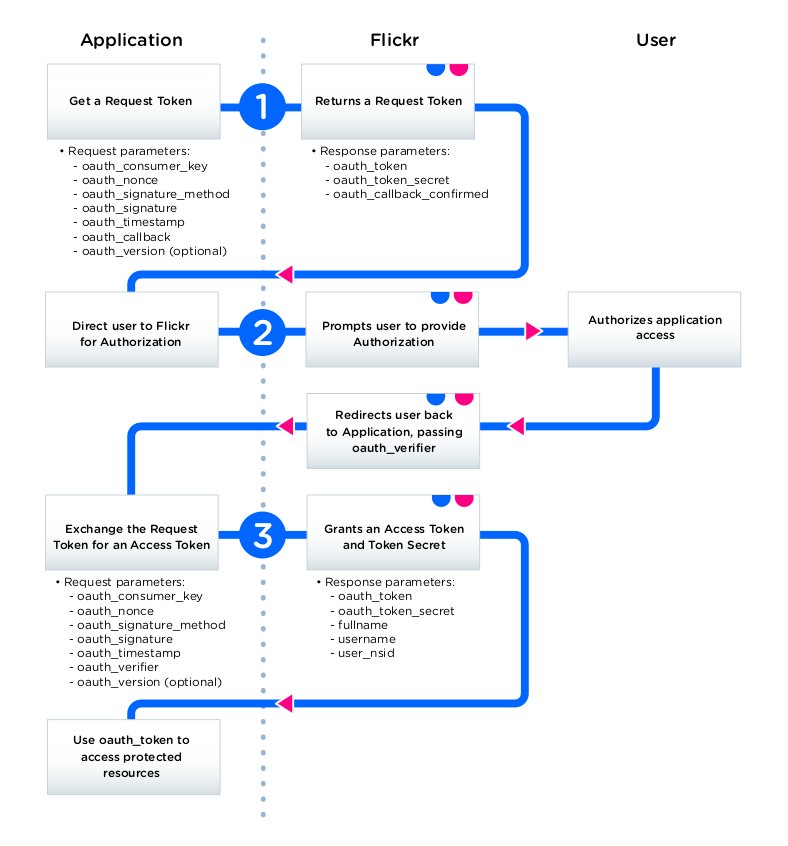
Flickr OAuth Authorization Flow
Signing Requests
You must sign all requests to the Flickr API. Currently, Flickr only supports HMAC-SHA1 signature encryption.
First, you must create a base string from your request. The base string is constructed by concatenating the HTTP verb, the request URL, and all request parameters sorted by name, using lexicograhpical byte value ordering, separated by an '&'.
Use the base string as the text
and the key
is the concatenated values of the Consumer Secret and Token Secret, separated by an '&'.
For example, using the following URL:
https://www.flickr.com/services/oauth/request_token ?oauth_nonce=89601180 &oauth_timestamp=1305583298 &oauth_consumer_key=653e7a6ecc1d528c516cc8f92cf98611 &oauth_signature_method=HMAC-SHA1 &oauth_version=1.0 &oauth_callback=http%3A%2F%2Fwww.example.com
Would have a base string that looks like the following:
GET&https%3A%2F%2Fwww.flickr.com%2Fservices%2Foauth%2Frequest_token&oauth_callback%3Dhttp%253A%252F%252Fwww.example.com%26oauth_consumer_key%3D653e7a6ecc1d528c516cc8f92cf98611%26oauth_nonce%3D95613465%26oauth_signature_method%3DHMAC-SHA1%26oauth_timestamp%3D1305586162%26oauth_version%3D1.0
Which would generate the following signature:
7w18YS2bONDPL%2FzgyzP5XTr5af4%3D
Getting a Request Token
Request Token URL:
https://www.flickr.com/services/oauth/request_token
The first step to obtaining authorization for a user is to get a Request Token using your Consumer Key. This is a temporary token that will be used to authenticate the user to your application. This token, along with a token secret, will later be exchanged for an Access Token.
The following is an example URL request for a request token:
https://www.flickr.com/services/oauth/request_token ?oauth_nonce=95613465 &oauth_timestamp=1305586162 &oauth_consumer_key=653e7a6ecc1d528c516cc8f92cf98611 &oauth_signature_method=HMAC-SHA1 &oauth_version=1.0 &oauth_signature=7w18YS2bONDPL%2FzgyzP5XTr5af4%3D &oauth_callback=http%3A%2F%2Fwww.example.com
Flickr returns a response similar to the following:
oauth_callback_confirmed=true &oauth_token=72157626737672178-022bbd2f4c2f3432 &oauth_token_secret=fccb68c4e6103197
Getting the User Authorization
User Authorization URL:
https://www.flickr.com/services/oauth/authorize
After getting the Request Token, your application needs to present the Flickr authorization page to the user, where they will be asked to give permission to your application to access their data. The authorization page will present the user with a list of permissions your application is requesting, as defined in the authentication flow settings.
Additionally, you can pass the optional perms=
parameter, asking for read
, write
, or delete
privileges. This parameter will override the setting defined in your application's authentication flow.
After authorization is complete, Flickr will redirect the user back to your application using the oauth_callback
specified with your Request Token.
The following is an example authorization URL:
https://www.flickr.com/services/oauth/authorize ?oauth_token=72157626737672178-022bbd2f4c2f3432
The following is an example callback after authorization:
http://www.example.com/ ?oauth_token=72157626737672178-022bbd2f4c2f3432 &oauth_verifier=5d1b96a26b494074
Exchanging the Request Token for an Access Token
Access Token URL:
https://www.flickr.com/services/oauth/access_token
After the user authorizes your application, you can exchange the approved Request Token for an Access Token. This Access Token should be stored by your application, and used to make authorized requests to Flickr.
The following is an example of a request for an Access Token:
https://www.flickr.com/services/oauth/access_token ?oauth_nonce=37026218 &oauth_timestamp=1305586309 &oauth_verifier=5d1b96a26b494074 &oauth_consumer_key=653e7a6ecc1d528c516cc8f92cf98611 &oauth_signature_method=HMAC-SHA1 &oauth_version=1.0 &oauth_token=72157626737672178-022bbd2f4c2f3432 &oauth_signature=UD9TGXzrvLIb0Ar5ynqvzatM58U%3D
Flickr returns a response similar to the following:
fullname=Jamal%20Fanaian &oauth_token=72157626318069415-087bfc7b5816092c &oauth_token_secret=a202d1f853ec69de &user_nsid=21207597%40N07 &username=jamalfanaian
Calling the Flickr API with OAuth
After an Access Token has been granted to your application, you can make authenticated requests to the Flickr API. Flickr requires HMAC-SHA1 encryption because all requests are being made insecurely using HTTP.
The following is an example of a request made to the flickr.test.login API:
https://www.flickr.com/services/rest ?nojsoncallback=1 &oauth_nonce=84354935 &format=json &oauth_consumer_key=653e7a6ecc1d528c516cc8f92cf98611 &oauth_timestamp=1305583871 &oauth_signature_method=HMAC-SHA1 &oauth_version=1.0 &oauth_token=72157626318069415-087bfc7b5816092c &oauth_signature=dh3pEH0Xk1qILr82HyhOsxRv1XA%3D &method=flickr.test.login
Flickr returns a response similar to the following:
{"user":{"id":"21207597@N07", "username":{"_content":"jamalfanaian"}}, "stat":"ok"}